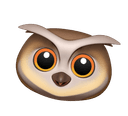
Flutter Flame tutorial with examples
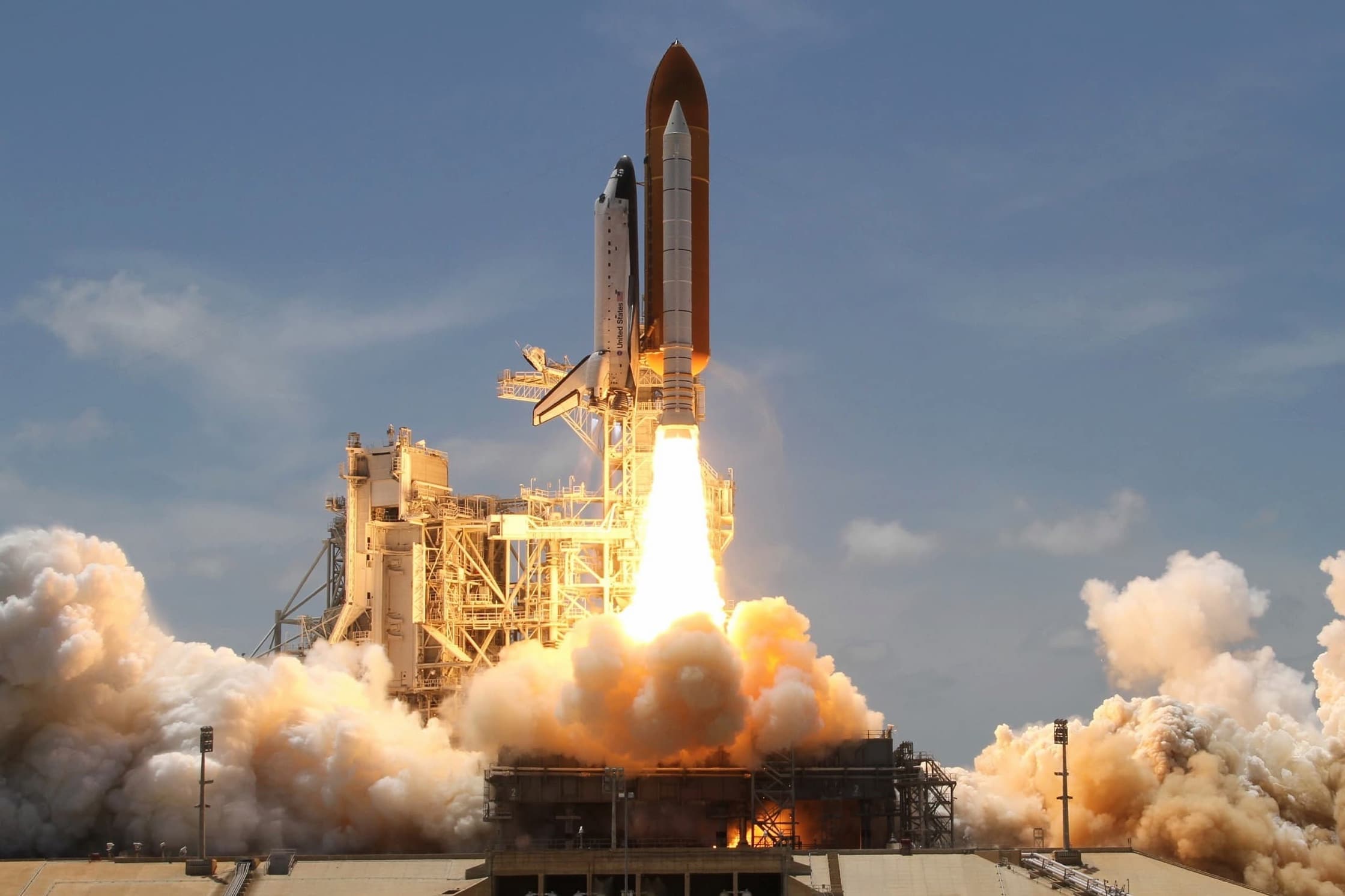
Getting Started
Flutter Flame is a powerful 2D game engine built on top of Flutter, allowing developers to create cross-platform mobile games and interactive experiences. If you're a complete beginner interested in game development and want to explore the world of Flutter Flame, you're in the right place. In this article, we'll guide you through the process of getting started with Flutter Flame and provide easy examples to help you grasp the fundamentals.
Pre-requisites
Before diving into Flutter Flame, make sure you have the following prerequisites in place:
Basic Understanding of Flutter: Familiarity with Flutter's basic concepts will be beneficial, though not mandatory. If you're new to Flutter, you can check out the official Flutter documentation and complete their beginner's tutorial.
Flutter SDK: Ensure you have the Flutter SDK installed on your development machine. You can download it from the official Flutter website and follow the installation instructions.
Code Editor: Choose a code editor or IDE of your preference. Popular choices include Visual Studio Code, Android Studio, and IntelliJ IDEA, with Flutter plugins installed.
Setting up a new project
Assuming you have Flutter set up on your machine, follow these steps to create a new Flutter project:
1. Open your terminal or command prompt and navigate to the directory where you want to create the project.
2. Run the following command to create a new Flutter project:flutter create my_game_project
3. Change the directory to the newly created project:cd my_game_project
Integrating Flutter Flame
Now that you have a basic Flutter project, you can integrate Flutter Flame into it. Follow these steps to add the necessary dependencies:
1. Open the pubspec.yaml
file in your project.
2. Under the dependencies
section, add the following line:flame: ^1.8.0
3. Save the file and run the following command to fetch and update the dependencies:flutter pub get
This loads and installs all dependencies.
Create a simple game
Now that you have Flutter Flame set up in your project, let's create a simple game. In this example, we'll create a basic "Flappy Bird" clone. This game will involve a bird flying through a series of pipes, and the player's task will be to keep the bird flying by tapping the screen.
Replace the code in lib/main.dart
with the following:
import 'package:flutter/material.dart';
import 'package:flame/game.dart';
import 'package:flame/flame.dart';
import 'package:flame/gestures.dart';
import 'package:flutter/gestures.dart';
void main() {
runApp(GameWidget());
}
class Bird {
final Game game;
Rect birdRect;
Paint birdPaint;
Bird(this.game) {
birdRect = Rect.fromLTWH(
game.size.width / 2 - 25,
game.size.height / 2 - 25,
50,
50,
);
birdPaint = Paint()..color = Colors.blue;
}
void render(Canvas canvas) {
canvas.drawRect(birdRect, birdPaint);
}
void update(double t) {}
}
class FlappyBirdGame extends FlameGame with TapDetector {
Bird bird;
@override
void onTapDown(TapDownDetails details) {
bird.fly();
}
@override
void update(double t) {
bird.update(t);
super.update(t);
}
@override
void render(Canvas canvas) {
super.render(canvas);
bird.render(canvas);
}
@override
void resize(Size size) {
super.resize(size);
bird = Bird(this);
}
}
class GameWidget extends StatelessWidget {
@override
Widget build(BuildContext context) {
Flame.device.fullScreen();
return MaterialApp(
home: Scaffold(
body: SafeArea(
child: FlappyBirdGame().widget,
),
),
);
}
}
In this code, we've created a simple game with a Bird
class and a FlappyBirdGame
class. The Bird
class represents the player's character (the bird), and the FlappyBirdGame
class is our game itself, handling the game loop and user input.