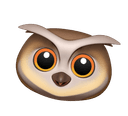
How to build a mobile game
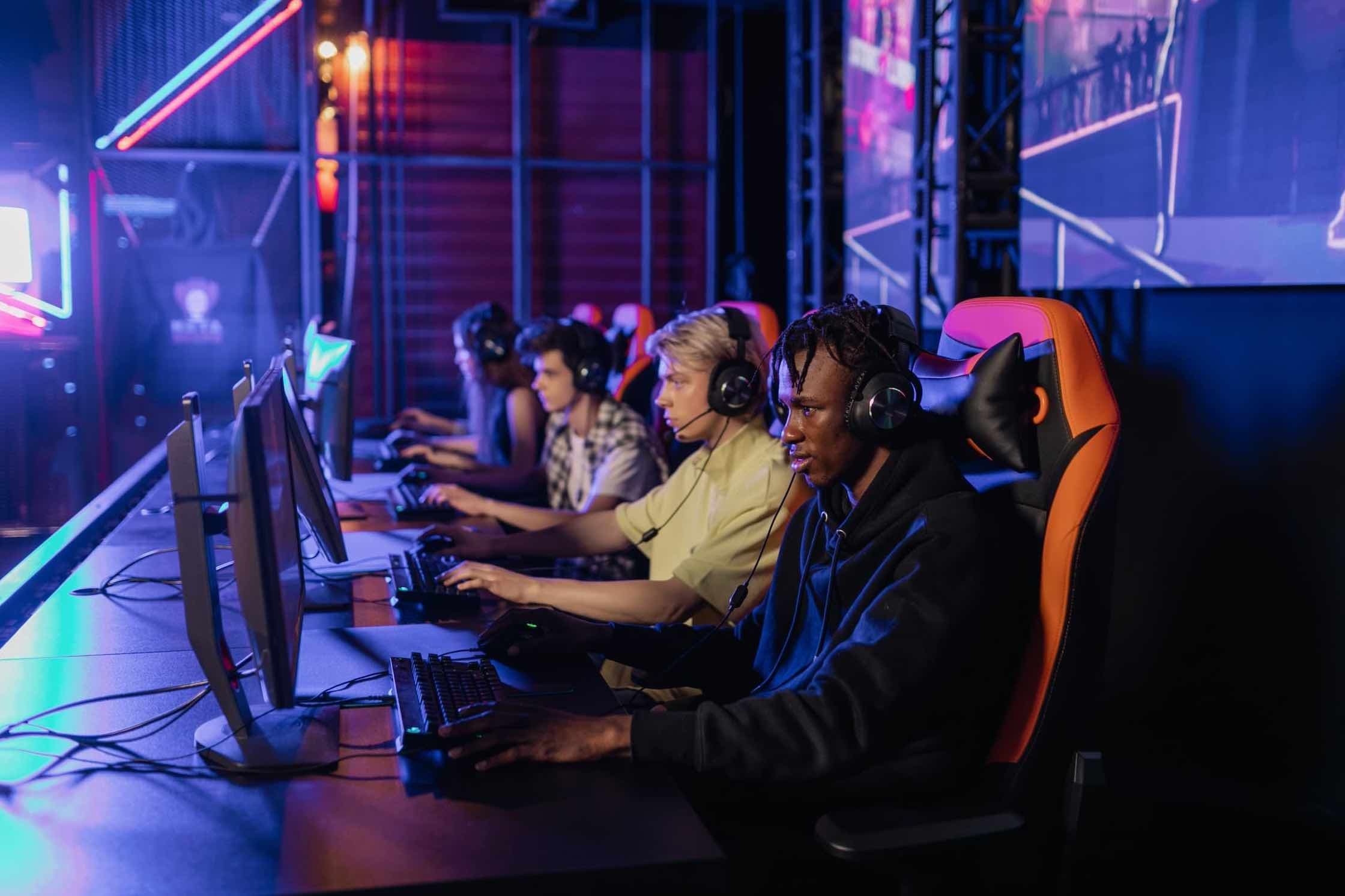
Introduction
In today's digital era, mobile gaming has become increasingly popular. If you've ever wondered how to create your own mobile game, you're in the right place! In this tutorial, we'll explore Flame, an open-source Flutter game engine that allows you to develop games for both iOS and Android platforms. We'll guide you through the process of building a simple mobile game using Flame and provide you with some sample code to get started. Let's dive in!
Prerequisites
Before we get started, make sure you have the following prerequisites:
1. Flutter SDK
Install Flutter by following the official documentation at https://flutter.dev/docs/get-started/install.
2. Dart programming language
Make sure you have Dart SDK installed, which comes bundled with Flutter.
3. Text editor or IDE
Choose a text editor or integrated development environment (IDE) of your preference, such as Visual Studio Code or Android Studio.
Step 1: Set Up a New Flutter Project
To begin, create a new Flutter project by running the following command in your terminal:
flutter create my_game
cd my_game
Step 2: Add Flame Dependency
Open the pubspec.yaml
file in your project and add the Flame dependency:
dependencies:
flutter:
sdk: flutter
flame: ^1.8.0
Save the file and run flutter pub get
in the terminal to fetch the Flame package.
Step 3: Create the Game Class
Create a new file called game.dart
in the `lib` directory and define the game class. This class will extend the FlameGame
class provided by the Flame package:
import 'package:flame/game.dart';
class MyGame extends FlameGame {
@override
Future<void> onLoad() async {
// Game initialization code
}
@override
void update(double dt) {
// Game logic code
}
@override
void render(Canvas canvas) {
// Game rendering code
}
}
Step 4: Initialize the Game
Open the main.dart
file and modify the default MyApp
widget to initialize your game:
import 'package:flutter/material.dart';
import 'package:flame/util.dart';
import 'package:flutter/services.dart';
import 'game.dart';
void main() async {
WidgetsFlutterBinding.ensureInitialized();
Util flameUtil = Util();
await flameUtil.fullScreen();
await flameUtil.setOrientation(DeviceOrientation.portraitUp);
MyGame game = MyGame();
runApp(game.widget);
}
Step 5: Implement Game Logic
In the game.dart
file, add your game logic inside the update
and render
methods. For this example, we'll create a simple game where a player sprite moves across the screen:
import 'package:flame/sprite.dart';
class MyGame extends FlameGame {
Sprite playerSprite;
double playerX = 0;
double playerY = 0;
@override
Future<void> onLoad() async {
playerSprite = await Sprite.load('player.png');
}
@override
void update(double dt) {
playerX += dt * 100; // Move player horizontally
if (playerX > size.x) playerX = 0; // Wrap around to the start
playerY += dt * 50; // Move player vertically
if (playerY > size.y) playerY = 0; // Wrap around to the top
}
@override
void render(Canvas canvas) {
playerSprite.render(canvas, position: Vector2(playerX, playerY));
}
}
Step 6: Run the Game
Save your changes and run the game using the flutter run
command in the terminal. Make sure you have a mobile device connected or an emulator running. You should see your game running on the device!
Conclusion
Congratulations! You've successfully built a simple mobile game using the Flame game engine in Flutter. Flame provides a wide range of features and tools to develop captivating mobile games. Feel free to explore the Flame documentation to learn more about advanced features and techniques for game development. Now, go ahead and unleash your creativity to build the next great mobile game!
Note: Remember to credit the authors of any assets you use in your game, such as sprites or sounds, as per their licensing terms.